This method is a simple way to send an email with WebClient.
- Create a function with these fields:
- toEmail: Recipient’s email
- fromEmail: Sender’s email
- subject: Subject from the email
- body: Message part from the email
- attachmentFileLocation(OPTIONAL): path from the file we will send as attachment
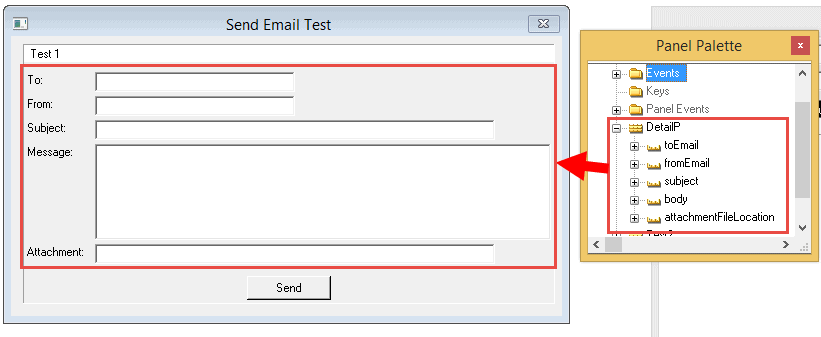
2. Add a source code object call “sendEmail” to your function.
3. Add the following fields as parameters to the “sendEmail” source code:
4. Copy the following code and paste it into the “sendEmail” source code:
import java.util.*; import javax.mail.*; import javax.mail.internet.*; import javax.activation.*; // Recipient's email String toEmail = & (1: ).toString(); // Sender's email ID String fromEmail = & (2: ).toString(); // Set email host String hostEmail = "YOUR EMAIL HOST"; // Get system properties Properties propertiesEmail = System.getProperties(); // Setup mail server propertiesEmail.setProperty("mail.smtp.host", hostEmail); // Get the default Session object. Session sessionEmail = Session.getDefaultInstance(propertiesEmail); try { // Create a default MimeMessage object. MimeMessage message = new MimeMessage(sessionEmail); // Set From message.setFrom(new InternetAddress(fromEmail)); // Set To message.addRecipient(Message.RecipientType.TO, new InternetAddress(toEmail)); // Set Subject message.setSubject( & (3: ).toString()); if ( & (5: ).toString().length() != 0) { // Create the message part BodyPart messageBodyPart = new MimeBodyPart(); // Fill the message messageBodyPart.setText( & (4: ).toString()); // Create a multipart message Multipart multipartEmail = new MimeMultipart(); // Set text message part multipartEmail.addBodyPart(messageBodyPart); // Part two is attachment messageBodyPart = new MimeBodyPart(); String filenameEmail = & (5: ).toString().replace('\\', '/'); DataSource sourceEmail = new FileDataSource(filenameEmail); messageBodyPart.setDataHandler(new DataHandler(sourceEmail)); messageBodyPart.setFileName(filenameEmail); multipartEmail.addBodyPart(messageBodyPart); // Send the complete message parts message.setContent(multipartEmail); } else { // Send the message message.setText( & (4: ).toString()); } // Definition of supported File Types MailcapCommandMap mc = (MailcapCommandMap) CommandMap.getDefaultCommandMap(); mc.addMailcap("text/html;; x-java-content-handler=com.sun.mail.handlers.text_html"); mc.addMailcap("text/xml;; x-java-content-handler=com.sun.mail.handlers.text_xml"); mc.addMailcap("text/plain;; x-java-content-handler=com.sun.mail.handlers.text_plain"); mc.addMailcap("multipart/*;; x-java-content-handler=com.sun.mail.handlers.multipart_mixed"); mc.addMailcap("message/rfc822;; x-java-content-handler=com.sun.mail.handlers.message_rfc822"); // Send message CommandMap.setDefaultCommandMap(mc); Transport.send(message); System.out.println("Sent message successfully...."); } catch (MessagingException mex) { mex.printStackTrace(); }
5. Change the value from the variable “hostEmail” to your host email.
6. Add a button called “Send” and a logical event called “sendEmail” in your function.
7. In the Action Diagram for the event “sendEmail” call the source code you created.
8. Generate your function and test it.
This is a simple example of how send mails with WebClient. Remember that you can modify the source code and function as you need.